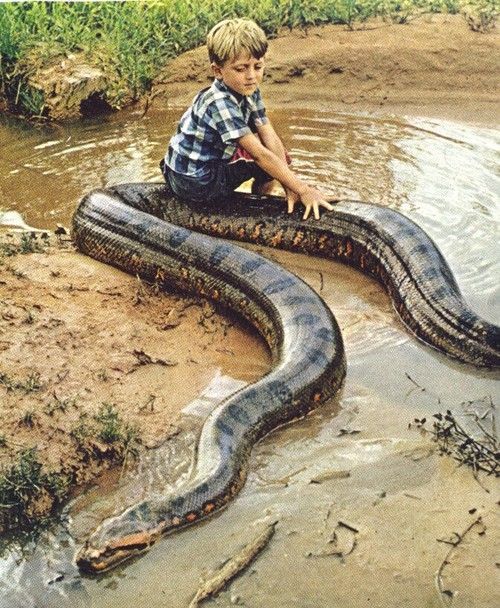
Python — The swiss army knife Part 2
For a quick recap:
- List
- a_list = [1,2,3,4,‘hello’]
- Tuple
- a_tuple = (1,2,3,4,‘hello’)
- Dictionary
- a_dict = {‘Name’:‘Puneet’, ‘Age’:28}
A tuple
is an immutable data structure while list
and dictionary
are mutable data structure.
Table of Contents
Slicing a list or a tuple
Slicing and subsetting a list
or a tuple
is same as selecting characters from a string. For example:
a = ['my', 'name', 'is', 'anthony', 'gonsalves']
print(a[0], a[-1], a[:-1], a[1:3], sep='\n')
## my
## gonsalves
## ['my', 'name', 'is', 'anthony']
## ['name', 'is']
b = (1,2,3,4,5,6)
print (b[-1], b[2:4], sep='\n')
## 6
## (3, 4)
A list/tuple
can contain many lists/tuples
as in nested lists/tuples
.A list
can contain tuples
as well as a tuple
can contain many lists
. Por ejemplo
nested_tuple = (1,2,3, [6,7])
nested_list = [1,2,[5,6,7],[8,9]]
print (nested_list[2], nested_list[1:3], sep='\n')
## [5, 6, 7]
## [2, [5, 6, 7]]
Iterating over a list, tuple and dictionary
Iterating over items in a list
, tuple
or dictionary
is achieved using for loop
. For example
list_of_fruits = ['orange', 'banana', 'apple', 'papaya']
for i in list_of_fruits:
print (i)
## orange
## banana
## apple
## papaya
Similarly for tuples
tuple_of_students = ('ravi', 'jack', 'ram', 'ronald')
for i in tuple_of_students:
print (i)
## ravi
## jack
## ram
## ronald
To iterate over a dictionary
,
dict_of_fruits = {'Apple':3, 'Orange':2, 'Banana':8}
for i, j in dict_of_fruits.items():
print (i,j)
## Apple 3
## Orange 2
## Banana 8
There are many more helpful iteration constructs which can be really fast and helpful.
Notice that whenever we write iteration constructs or control statements, we end the statement with a colon and indent the code. Indenting the code in python is forced. Without indenting, the code will throw up error. In principle, decide for one rule of indenting and follow it in all your coding statements. Indenting can be done for 1, 2 or any number of spaces or tab. Generally 4 space rule is followed. This forced indenting makes the code look cleaner and more readable.
Some helpful constructs in python
Enumerate
enumerate
is helpful when you want to iterate over a sequence of list/tuple/dictionary/string
such that you wish to access both the index and the item at the same time. For example
list_of_fruits = ['Apple','Orange','Banana','Grapes','Watermelon']
for i, val in enumerate(list_of_fruits):
print (i,val)
## 0 Apple
## 1 Orange
## 2 Banana
## 3 Grapes
## 4 Watermelon
Zip
zip
function is helpful when you want to simultaneously iterate over multiple lists/tuples/dictionaries
me = [1,2,3,4]
you = [5,6,7,8,9]
for i, j in zip(me,you):
print (i,j)
## 1 5
## 2 6
## 3 7
## 4 8
Notice that if list/tuple/dictionaries
are not of same length then the smaller length is iterated
There are many functions available in python which are helpful in many ways.
a = [1,3,5,2,4]
print (len(a), min(a), max(a))
## 5 1 5
Conditional statements
if-else
a = [1,2,3,4]
for i in a:
if i>2:
print (i)
else:
print ('digit less than 2')
## digit less than 2
## digit less than 2
## 3
## 4
if-elif-else
a = [1,2,3,4,5,6,7,8,9]
for i in a:
if i<3:
print ('less than 3')
elif i<6:
print ('less than 6')
else:
print (i)
## less than 3
## less than 3
## less than 6
## less than 6
## less than 6
## 6
## 7
## 8
## 9
while
x = 0
while x<5:
print (x)
x = x + 1
## 0
## 1
## 2
## 3
## 4
Break and Continue
Sometimes we need to stop the iteration if a condition is met and break out of the loop. Then we use break
statement.
a = [10,14,15,18,19,21,25,28]
for i, val in enumerate(a):
if i > 3:
break
print (val)
## 10
## 14
## 15
## 18
Sometimes during the iteration we want that if a condition is satisfied, the operation within the loop is skipped. Then we use continue
statement.
a = [1,2,3,4,6,8,9,10]
for val in a:
if val%2==0:
continue
print (val)
## 1
## 3
## 9
Range function
import numpy
arr = numpy.arange(10)
for i in range(len(arr)):
print (i)
## 0
## 1
## 2
## 3
## 4
## 5
## 6
## 7
## 8
## 9
a = [1,2,3,4,5,6,7,8,9,10,11,12,13,14]
for i in range(0,len(a),3):
print (a[i])
## 1
## 4
## 7
## 10
## 13